Handle Websocket API Frontend Implementation
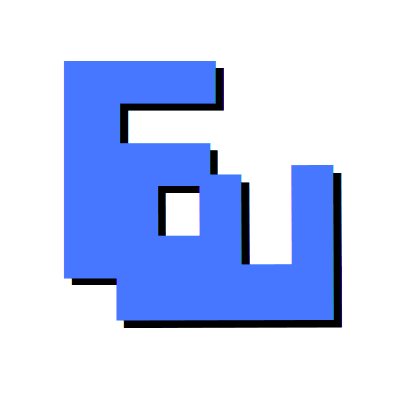
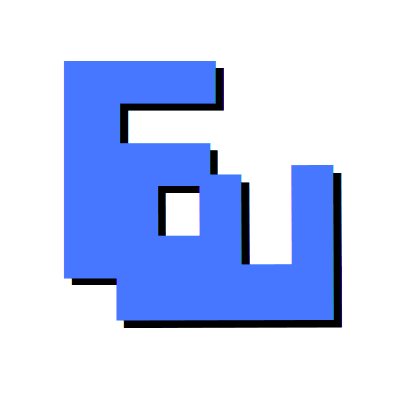
Handle Websocket with Websocket API in Javascript. The browser API has a function for communicate between server and user browser. What we must provide the API for creating and managing a WebSocket connection to a server, as well as for sending and receiving data on the connection. There are some kay what we must know.
WebSocket
The primary interface for connecting to a WebSocket server and then sending and receiving data on the connection.
OpenEvent
The event sent by the WebSocket object when the connection open.
CloseEvent
The event sent by the WebSocket object when the connection closes.
MessageEvent
The event sent by the WebSocket object when a message is received from the server.
For Example Implementation:
TLDR; Scroll to bottom for the complete code
1const wsConnection = new WebSocket(`${wsURL}`);
the example above are for the initialization of creating a websocket object.
1wsConnection.onopen = function () {
2 const params = { key: 'value' }
3 wsConnection.send(
4 JSON.stringify(params)
5 );
6 setTimeout(heartbeat, 1000); // optional
7};
when websocket are open this will sending the params data by calling send method to the server. In the example the params data was an Object. So, we must stringify the data so can be received by the server. Also there was heartbeat function that will be called after 1 second. This function will be decribed in the next.
1function heartbeat() {
2 if (!wsConnection || wsConnection.readyState !== 1) {
3 return;
4 }
5 const params = { key: 'value'};
6
7 wsConnection.send(JSON.stringify(params));
8 setTimeout(heartbeat, 3000);
9}
The heartbeat function are recursively calling and send the data every 3 second (in code above). First condition was checking the connection, if there are not any connection so this function will stop.
1wsConnection.onmessage = function (event) {
2 try {
3 var data = JSON.parse(event.data);
4
5 // code that process the response data
6 } catch (e) {
7 //console.error('got non json data', event.data, e);
8 }
9}
The onmessage function are receiving response from the server. After that, we can produce the response data on the inside try code, and if there was error will catch and handle the error.
1wsConnection.onclose = function (e) {
2 if (e.code != 1000) {
3 closeWS();
4 } else {
5 setTimeout(function () {
6 initWS();
7 }, 1000);
8 }
9};
The next step is handling websocket onclose. When an Websocket closed that should be close by error connection or own close. If we don't want to close yet, we can call init function again.
1function closeWS() {
2 if (wsConnection) {
3 wsConnection.onclose = function () {
4 wsConnection = null;
5 };
6 wsConnection.close();
7 }
8}
In The closeWS function we can close the connection by calling close function. Also make the object null. For the example full code are below.
1export let wsURL;
2
3let wsConnection;
4let wsTries = 5; // max websocket can try to init
5let timeout = 1000;
6
7wsURL = 'http://url';
8
9export function initWS() {
10 if (wsTries <= 0) {
11 console.error('unable to estabilish WS after 5 tries!');
12 wsConnection = null;
13 wsTries = 5;
14 return;
15 }
16 //Don't open a new websocket if it already exists. Figure out a better way for event filtering
17 if (wsConnection) {
18 return;
19 }
20 const params = { key: 'value' }
21
22 wsConnection = new WebSocket(`${wsURL}`);
23 wsConnection.onopen = function () {
24 wsConnection.send(
25 JSON.stringify(
26 Object.assign(params)
27 )
28 );
29 setTimeout(heartbeat, 1000);
30 };
31
32 // Log errors
33 wsConnection.onerror = function (error) {
34 wsTries--;
35 console.error('WebSocket Error ', error);
36 };
37
38 // Log messages from the server
39 wsConnection.onmessage = function (event) {
40 try {
41 var data = JSON.parse(event.data);
42 closeWS();
43 } catch (e) {
44 //console.error('got non json data', event.data, e);
45 }
46 };
47 wsConnection.onclose = function (e) {
48 if (e.code != 1000) {
49 closeWS();
50 } else {
51 setTimeout(function () {
52 initWS();
53 }, timeout);
54 }
55 };
56}
57
58function closeWS() {
59 if (wsConnection) {
60 wsConnection.onclose = function () {
61 wsConnection = null;
62 };
63 wsConnection.close();
64 WS_ACTIVE.set(false);
65 }
66}
67
68function heartbeat() {
69 if (!wsConnection || wsConnection.readyState !== 1) {
70 return;
71 }
72 const params = { key: 'value' }
73 wsConnection.send(JSON.stringify(params));
74 setTimeout(heartbeat, 3000);
75}
76
77
Hope this code will help you in implementing websocket in the future. Thank you for reading :)